Adding a product for the online shop is a straightforward process. Just enter the product information and hit the 'Add Product' button. When adding a product we will require these information :
- Category
- Product name
- Description
- Price
- Quantity in stock
- Image
Not much difference from the add category form.
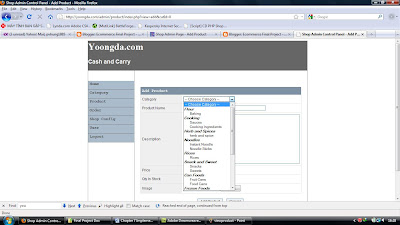
On top of the form the admin can see the category combo box. I build this so that the admin can only select the second level category. This is to ensure that all product are added on the second level category and not put in the top level category by mistake.
The one responsible to build the list is the buildCategoryOptions() function in admin/library/functions.php. Below is the snippet for the code that build the combo box. Just in case I will need the ability to add some product to the first level category you can replace the buildCategoryOptions() with the commented function code written below it.
function buildCategoryOptions($catId = 0)
{
$sql = "SELECT cat_id, cat_parent_id, cat_name
FROM tbl_category
ORDER BY cat_id";
$result = dbQuery($sql) or die('Cannot get Product. ' . mysql_error());
$categories = array();
while($row = dbFetchArray($result)) {
list($id, $parentId, $name) = $row;
if ($parentId == 0) {
// we create a new array for each top level categories
$categories[$id] = array('name' => $name, 'children' => array());
} else {
// the child categories are put int the parent category's array
$categories[$parentId]['children'][] = array('id' => $id, 'name' => $name);
}
}
// build combo box options
$list = '';
foreach ($categories as $key => $value) {
$name = $value['name'];
$children = $value['children'];
$list .= "";
foreach ($children as $child) {
$list .= ";
if ($child['id'] == $catId) {
$list.= " selected";
}
$list .= ">{$child['name']}rn";
}
$list .= "";
}
return $list;
}
The product quantity is limited to 65,535 since in the table definition I only use smallint(5) . I find this number adequate for most online shops. But if do carry more than that amount for an item just change the data type to something bigger like mediumint(8).The product image you need to supply is the large size product image which will be shown in the product detail page. The script will generate a thumbnail for it to be shown in the product browsing page. I define the maximum image size and the thumbnail size in config.php
I need to restrict the image size so it won't destroy the site layout. Imagine that if the image is 1000 pixels wide and 2000 pixels high. It will make the product detail page look awful. Image resizing can be turned on or off. If you set LIMIT_PRODUCT_WIDTH to false on config.php the script will just upload the image without worying about it's size. It's not recommended though.// some size limitation for the category
// and product images
// all category image width must not
// exceed 75 pixels
define('MAX_CATEGORY_IMAGE_WIDTH', 75);
// do we need to limit the product image width?
// setting this value to 'true' is recommended
define('LIMIT_PRODUCT_WIDTH', true);
// maximum width for all product image
define('MAX_PRODUCT_IMAGE_WIDTH', 300);
// the width for product thumbnail
define('THUMBNAIL_WIDTH', 75);
if (!get_magic_quotes_gpc()) {
if (isset($_POST)) {
foreach ($_POST as $key => $value) {
$_POST[$key] = trim(addslashes($value));
}
}
if (isset($_GET)) {
foreach ($_GET as $key => $value) {
$_GET[$key] = trim(addslashes($value));
}
}
}
No comments:
Post a Comment